Create Customer/Location in C#
This section generically refers to physical locations where service may be delivered.
Multiple entities must be created to ensure the service location is ready for basic Work Order processing:
- WorkZone
- Customer
- Space
- Contact
Creating at least one Contact entity is recommended if your organization will be leveraging the power of the Corrigo Customer Portal.
First, use the WorkZoneCreateCommand to create the WorkZone entity.
A Work Zone is a grouping or parent entity for Customers, Work Orders, and Assets. It provides two important aspects for Work Order execution:
- defines the geographical context (physical location/postal address, time zone).
- defines the workflow configuration (specialty-based assignments, on-call lists, escalation lists, business hours, etc.)
Work Zone Creation Defaults
During Work Zone creation, globally configured Work Zone defaults (listed in the table) can be leveraged by setting the command attributes AssetTemplateId and SkipDefaultSettings to 0 and false respectively.
Work Zone Creation Defaults |
---|
Default template |
Default Team |
Use local business hours |
Use local holidays |
Use local emergency escalation |
Use local on-call schedule |
Work Order Digits |
Assignment dialog Users |
Unassigned Work Orders |
Only the minimal required elements to create a Work Zone are shown.
/* create WorkZone */
var workZone = new WorkZone {
DisplayAs = workZoneName,
Number = workZOneNumber,
WoNumberPrefix = woNumberPrefix,
TimeZone = timeZoneId
};
var results = corrigoService.Execute(
new WorkZoneCreateCommand {
WorkZone = workZone,
/* these 2 settings apply globally configured values
for the Work Zone properties listed in the table */
AssetTemplateId = assetTemplates[0].Id,
SkipDefaultSettings = false
}
);
/* newly created WorkZone Id */
var response = results as OperationCommandResponse;
workZone.Id = response.EntitySpecifier.Id;
Notice that data returned from the WorkZoneCreateCommand includes the WorkZone.Id value of the newly created Work Zone entity.
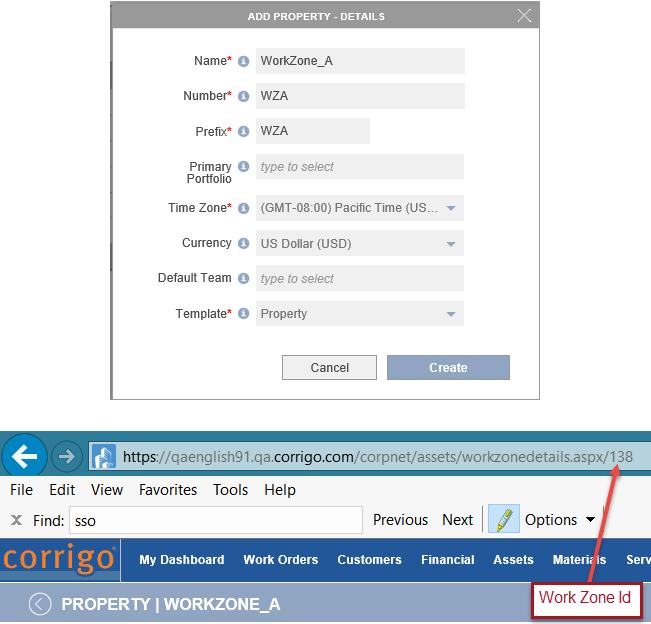
Creating a Work Zone
Next, use CreateCommand to create Customer entity, noting how the Id from the prior operation is used to reference the newly created Work Zone.
var customer = new Customer
{
/* newly created WorkZone Id from the prior step */
WorkZone = new WorkZone { Id = workZone.Id },
Name = customerName,
/* Customer Number must be unique */
TenantCode = tenantCode
};
var results = corrigoService.Execute(new CreateCommand { Entity = customer });
/* newly created Customer Id */
var response = results as OperationCommandResponse;
customer.Id = response.EntitySpecifier.Id;
Again, notice that Customer.Id value is present in the response to the operation.
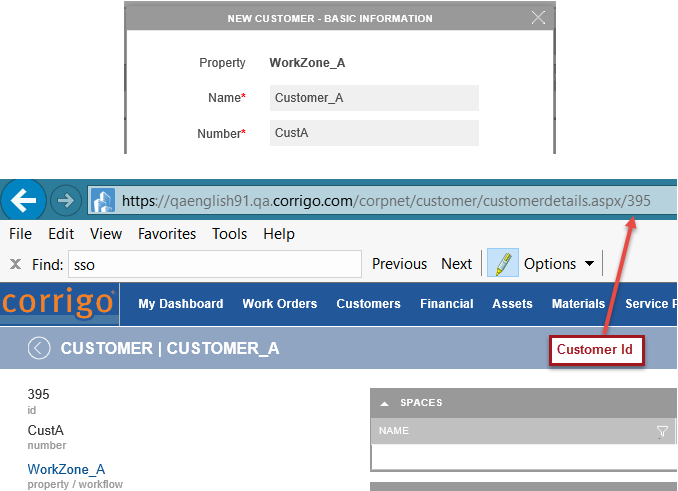
Creating a Cutsomer
A Space is the next entity to create. This entity uses an entity specific create command (SpaceCreateCommand) because to create a Space, multiple references are required including WorkZone, Customer and Asset Templates.
Asset templates are collections of labelled models, that exist in a hierarchical relationship with one another. A Space is essentially occupied by the Customer and is associated with a Unit asset template. The NewUnitSpecifier class is used to specify the asset template to be used in the Space creation command.
var command = new SpaceCreateCommand
{
/* newly created Customer Id from prior step to link Space to Customer */
CustomerId = customer.Id,
NewUnitSpecifier = new NewUnitSpecifier
{
UnitName = unitName,
/* asset template name */
UnitFloorPlan = unitFloorPlan,
StreetAddress = new Address2 { ActorTypeId = ActorType.CommLeaseSpace, }
}
};
var response = corrigoService.Execute(command);
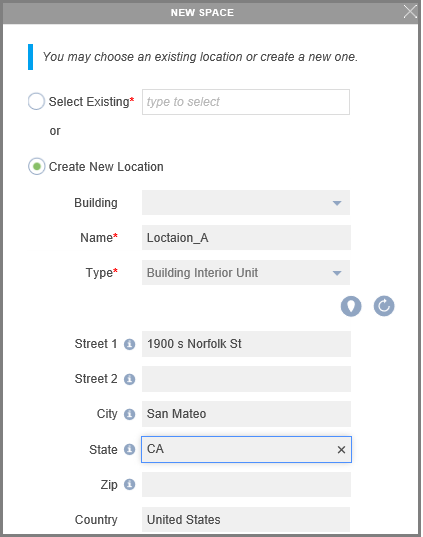
Creating a Space
Finally, use CreateCommand to create Contact entity.
/* create customer contact */
var contact = new Contact
{
/* newly created Customer Id from prior step to link Contact to Customer*/
CustomerId = customer.Id,
LastName = lastName,
/* login */
Username = userName,
Number = number
};
var resultData = service.Execute(new CreateCommand { Entity = contact });
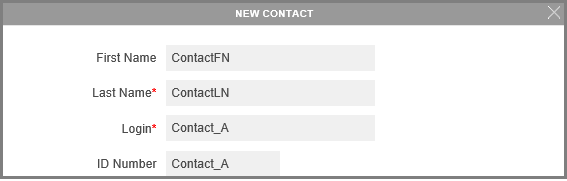
Creating a Contact
The new Customer is created.
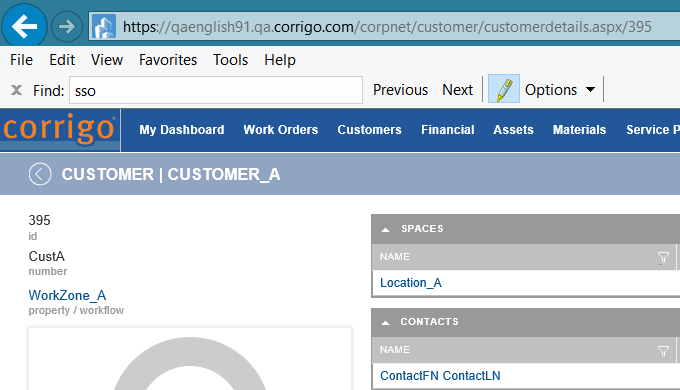
New Customer with associated Work Zone, Space, and Contact
Updated over 4 years ago