Create Work Order in C#
With a fully-defined Customer/Location, Work Order creation is possible. A WorkOrder entity is a very deep and rich structure, but we will focus only on the bare minimum here.
These children entities should be included:
- WoPriority
- SubType
- Task
- MainAsset
- Items collection
The MainAsset child entity can be retrieved through the Location entity. (Location refers to a specific location within the Asset tree).
Note
The values generated in the Create Customer/Location samples will be re-used in this sample.
First, use RetrieveMultiple to query the available WoPriority and SubType entities, in order to identify the Id values to be referenced in later operations.
/* retrive all Work Order Priorities */
var prioritiesList = corrigoService.RetrieveMultiple(
new QueryByProperty
{
EntityType = EntityType.WoPriority,
PropertySet = new AllProperties(),
Conditions = new PropertyValuePair[0]
}
);
/* retrieve all Work Order Sub Types */
var subTypesList = corrigoService.RetrieveMultiple(
new QueryByProperty
{
EntityType = EntityType.WorkOrderType,
PropertySet = new AllProperties(),
Conditions = new PropertyValuePair[0]
}
);
Next, use RetrieveMultiple to query the available Location entities for a specific Space using the name of the Space.
var locationList = corrigoService.RetrieveMultiple(
new QueryExpression
{
EntityType = EntityType.Location,
PropertySet = new AllProperties(),
Criteria = new FilterExpression
{
Conditions = new ConditionExpression[]
{
new ConditionExpression
{
PropertyName = "Name",
Operator = ConditionOperator.Equal,
/* provide Space name */
Values = new object[] {spaceName}
}
},
FiterOperator = LogicalOperator.Or
},
Orders = new[]
{
new OrderExpression
{
OrderType = OrderType.Descending,
PropertyName = "Id"
}
},
}
);
After selecting a Location Id from the above result set to represent the MainAsset, retrieve Tasks entities that are related to the Model of the MainAsset.
/* retrieve tasks for a specifc Model */
var list = service.RetrieveMultiple(
new QueryExpression
{
EntityType = EntityType.Task,
PropertySet = new AllProperties(),
Criteria = new FilterExpression
{
Conditions = new ConditionExpression[]
{
new ConditionExpression
{
PropertyName = "ModelId",
Operator = ConditionOperator.Equal,
/* specify Model Id of the MainAsset */
Values = new object[] {modelId}
}
},
FilterOperator = LogicalOperator.Or
},
Orders = new[]
{
new OrderExpression
{
OrderType = OrderType.Descending,
PropertyName = "Id"
}
},
}
);
Finally, assemble the WoCreateCommand with valid references to required child entities to create the Work Order.
var workOrder = new WorkOrder
{
Items = new[]
{
new WoItem
{
Asset = new Location { Id = spaceUnitSubAssetId },
Task = new Task { Id = taskId }
}
},
Customer = new Customer { Id = customerId },
/* Work Order type */
TypeCategory = WOType.Request,
SubType = new WorkOrderType { Id = subTypeId },
ContactAddress = new ContactInfo
{
Address = address,
AddrTypeId = ContactAddrType.Contact
}
};
var command = new WoCreateCommand
{
WorkOrder = workOrder,
ComputeAssignment = computeAssignment,
ComputeSchedule = computeSchedule,
SkipBillToLogic = false
};
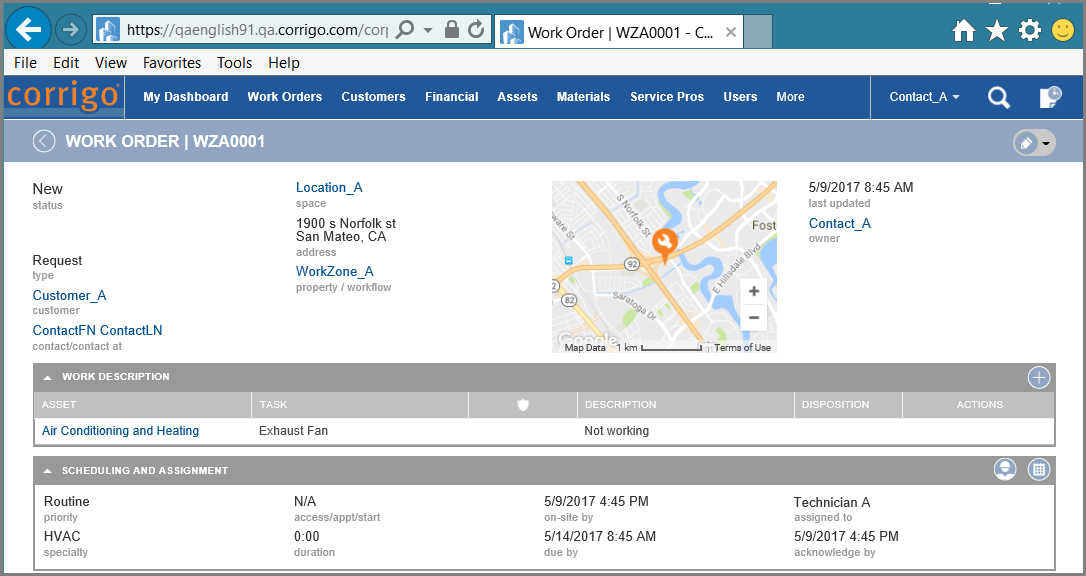
Updated almost 4 years ago